About: In this example we will see how to limit number of characters to be entered in a HTML textarea. To know more about textarea tag, click here.
Pre-requisites:
What if we would like to have limited number of characters that users can add in them? For that purpose we can use JQuery as a solution.
Solution:
HTML Code - Add the following code under body tag of your HTML:
JQuery Code - Add the following code under document.ready function in JQuery:
Conclusion: You will see that on top of textarea, the number of characters decreases when you enter any character in a textarea. You will also notice that once it reaches zero (0), it will not accept further characters in a textarea.
Note: If you are changing the value of variable "maxchars", don't forget to change the same in span tag. :)
Pre-requisites:
- Knowledge of HTML
- Knowledge of JQuery
What if we would like to have limited number of characters that users can add in them? For that purpose we can use JQuery as a solution.
Solution:
HTML Code - Add the following code under body tag of your HTML:
<span id="remaining">100</span> characters remaining.
<br />
<textarea></textarea>
JQuery Code - Add the following code under document.ready function in JQuery:
var maxchars = 100;
$('textarea').keyup(function () {
var textAreaLength = $(this).val().length;
$(this).val($(this).val().substring(0, maxchars));
textAreaLength = $(this).val().length;
remaining = maxchars - parseInt(textAreaLength);
$('#remaining').text(remaining);
});
Conclusion: You will see that on top of textarea, the number of characters decreases when you enter any character in a textarea. You will also notice that once it reaches zero (0), it will not accept further characters in a textarea.
Note: If you are changing the value of variable "maxchars", don't forget to change the same in span tag. :)
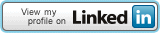